Beginners Android App Development: A Comprehensive Guide
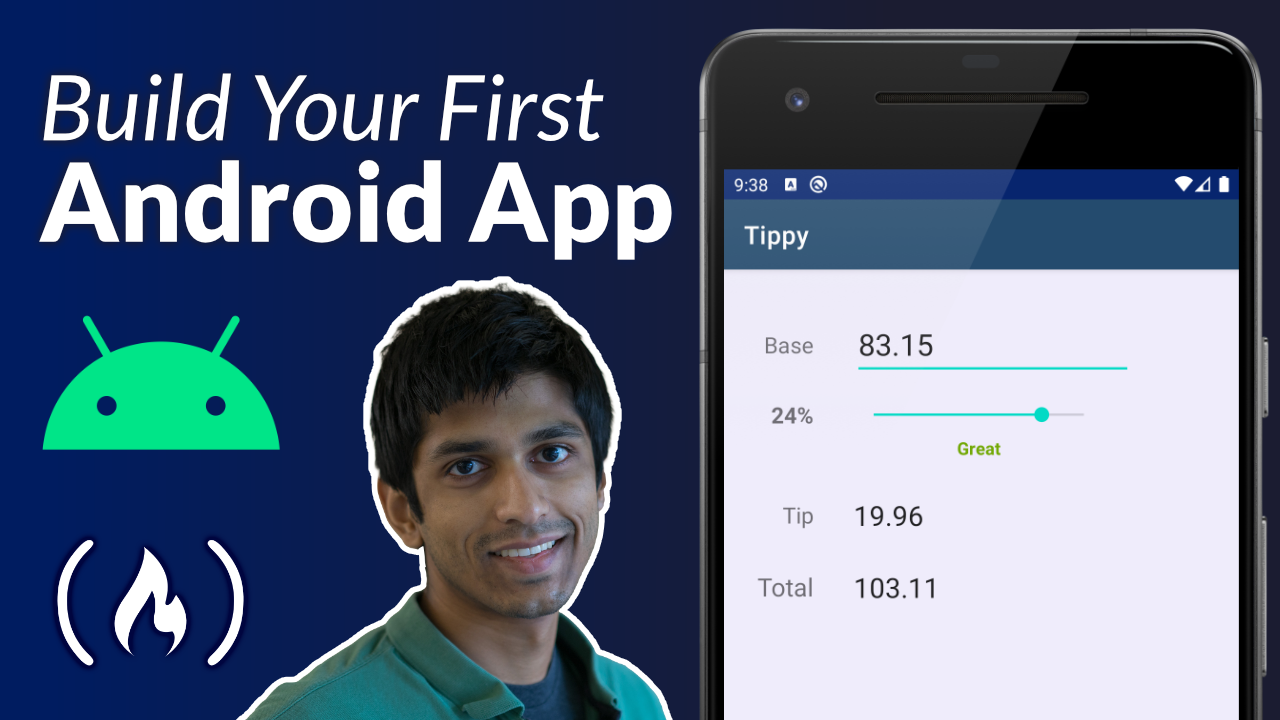
Are you interested in creating your own Android app but don't know where to start? Look no further! In this comprehensive guide, we will walk you through the process of beginner Android app development, providing you with all the essential knowledge and resources you need to get started on your app development journey.
Whether you have no prior coding experience or are a seasoned developer looking to expand your skills, this guide is designed to cater to all levels of expertise. From understanding the basics of Android app development to learning the necessary programming languages and tools, we have got you covered.
So, let's dive into the exciting world of Android app development and unlock your potential to create innovative and functional apps that can make a difference!
Understanding Android App Development
Android app development has become a prominent field in the tech industry, offering countless opportunities for developers. Before we delve into the technical aspects of creating Android apps, it's essential to understand what Android app development entails and why it has gained such popularity.
The Significance of Android App Development
Android, developed by Google, is the most widely used mobile operating system globally, powering millions of devices. The popularity of Android creates a massive demand for apps that cater to the diverse needs and preferences of users. By developing Android apps, you can reach a vast user base and potentially make a significant impact.
Additionally, Android app development allows you to tap into the ever-growing mobile market, which is constantly evolving and expanding. With the right skills and creativity, you can create apps that solve real-world problems, enhance user experiences, and even generate revenue through various monetization strategies.
Career Opportunities in Android App Development
Android app development offers a wide range of career opportunities for aspiring developers. As the demand for innovative and functional Android apps continues to rise, businesses are actively seeking talented developers to create and maintain their apps.
Whether you aspire to work for established companies, startups, or even freelance, Android app development can open doors to exciting job prospects. You can specialize in various areas of Android app development, such as UI/UX design, backend development, or even become an Android app consultant. The possibilities are endless!
Setting Up Your Development Environment
Before you embark on your Android app development journey, it's crucial to set up your development environment properly. This section will guide you through the necessary steps to install and configure the required software and tools, ensuring a smooth and efficient development process.
Installing Android Studio
Android Studio is the official integrated development environment (IDE) for Android app development. It provides a comprehensive set of tools and features that streamline the app creation process. To get started, visit the official Android Studio website and download the latest version compatible with your operating system.
Once the download is complete, run the installer and follow the on-screen instructions to install Android Studio. During the installation, make sure to select the appropriate components based on your project requirements. Android Studio will also install the necessary Android SDK (Software Development Kit) and other dependencies.
Configuring Android Virtual Device (AVD)
An Android Virtual Device (AVD) allows you to emulate and test your apps on different virtual devices without the need for physical devices. To configure an AVD, open Android Studio and navigate to the "AVD Manager" from the toolbar or the "Welcome to Android Studio" window.
In the AVD Manager, click on "Create Virtual Device" and select the device configuration you want to emulate. You can choose from various device profiles, each representing different screen sizes, resolutions, and hardware specifications. Once you have selected a device, click "Next" and follow the prompts to complete the AVD creation process.
Exploring Android Studio Interface
Now that you have Android Studio set up and an AVD configured, let's familiarize ourselves with the Android Studio interface. When you launch Android Studio, you will be greeted with a welcome screen that provides quick access to recent projects, tutorials, and other helpful resources.
The main interface of Android Studio consists of several panels and windows, each serving a specific purpose. The most prominent panel is the editor window, where you will write your code. On the left side, you will find the project navigator, displaying the project structure and files.
At the bottom, you will see the logcat window, which displays system logs and debugging information. On the top, there is a toolbar with various buttons for running, debugging, and building your app. The interface may appear overwhelming at first, but with practice, you will become familiar with its layout and functionality.
Understanding Android App Architecture
Android app architecture forms the foundation for building robust and scalable apps. In this section, we will explore the key components of Android app architecture, providing you with a solid understanding of how different elements work together to create functional and efficient apps.
Activities: The Building Blocks
In Android, an activity represents a single screen with a user interface. Activities serve as the building blocks of an app, allowing users to navigate between different screens and interact with the app's features and content. Understanding activities is crucial for creating app flows and managing user interactions.
Each activity is associated with a specific layout file, which defines the visual elements and user interface components of the screen. By combining multiple activities, you can create complex app structures and provide seamless user experiences.
Fragments: Reusable UI Components
Fragments are reusable UI components that represent a portion of an activity's user interface. Fragments allow you to build flexible and modular app designs, as they can be combined or replaced within activities based on different screen sizes, orientations, or other conditions.
By using fragments, you can create apps that adapt to various devices, such as smartphones and tablets, without duplicating code or layouts. Fragments have their lifecycle and can handle user input, making them powerful tools for creating dynamic and interactive app interfaces.
Layouts: Defining App UI
Layouts in Android define the structure and appearance of app screens. They are created using XML (eXtensible Markup Language), a markup language that allows you to describe the user interface elements and their properties. Understanding layouts is essential for designing visually appealing and user-friendly app interfaces.
Android provides a wide range of layout options, such as LinearLayout, RelativeLayout, and ConstraintLayout, each offering unique capabilities for organizing and positioning UI elements. By combining layouts and nesting them within activities and fragments, you can create complex and responsive app interfaces.
Views: Interactive UI Elements
Views are the fundamental building blocks of the user interface in Android. They represent interactive UI elements, such as buttons, text fields, images, and more. Each view has its properties and behaviors, allowing users to interact with the app and providing visual feedback.
Android offers a vast collection of pre-defined views, covering almost every type of user interface element you can imagine. By combining views, you can create rich and engaging app interfaces that cater to the specific requirements of your app.
Java Programming for Android
Java is the primary programming language used in Android app development. In this section, we will introduce you to the basics of Java programming, equipping you with the necessary knowledge to write code for your Android apps.
Variables and Data Types
Variables are essential for storing and manipulating data in a program. In Java, you need to declare variables before using them. The declaration specifies the variable's name and data type. Data types define the kind of data a variable can hold, such as integers, floating-point numbers, strings, and more.
For example, you can declare an integer variable named "age" as follows:
```int age;```
You can assign a value to the variable using the assignment operator (=):
```age = 25;```
Java provides various data types, including primitive types (int, double, boolean) and reference types (String, arrays, custom objects). Understanding data types is essential for efficient memory usage and performing operations on variables.
Control Structures: Making Decisions and Loops
Control structures allow you to control the flow of execution in your program based on specific conditions. Two fundamental control structures in Java are if-else statements and loops.
The if-else statement allows you to execute different blocks of code based on a condition. For example:
```javaint age = 18;
if (age >= 18) {System.out.println("You are eligible to vote.");} else {System.out.println("You are not eligible to vote yet.");}```
Loops, such as for loops and while loops, enable you to repeat a block of code multiple times. For example:
```javafor (int i = 1; i <= 5; i++) {System.out.println("Count: " + i);}```
Control structures are crucial for implementing decision-making logic and performing repetitive tasks in your Android apps.
Object-Oriented Programming (OOP) Concepts
Java is an object-oriented programming (OOP) language, which means it emphasizes the use of objects to represent real-world entities and their interactions. Understanding OOPconcepts is vital for creating well-structured and modular Android apps. Here are some key OOP concepts in Java:
Classes and Objects
In Java, a class is a blueprint for creating objects. It defines the properties (attributes) and behaviors (methods) that objects of that class will have. For example, you can create a class called "Car" with attributes such as "brand," "model," and methods like "startEngine" and "drive."
To create an object from a class, you use the "new" keyword:
```javaCar myCar = new Car();```
You can then access the object's attributes and invoke its methods using the dot notation:
```javamyCar.brand = "Toyota";myCar.startEngine();```
Inheritance
Inheritance allows you to create new classes based on existing classes, inheriting their attributes and behaviors. The existing class is called the superclass, while the new class is called the subclass. The subclass can add new attributes and behaviors or override the existing ones.
```javaclass Animal {void sound() {System.out.println("Animal makes a sound");}}
class Cat extends Animal {void sound() {System.out.println("Cat meows");}}```
In this example, the "Cat" class is a subclass of the "Animal" class. It overrides the "sound" method to provide a specific implementation for cats.
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It allows you to write code that can work with objects of different types, providing flexibility and extensibility in your programs.
```javaclass Animal {void sound() {System.out.println("Animal makes a sound");}}
class Cat extends Animal {void sound() {System.out.println("Cat meows");}}
class Dog extends Animal {void sound() {System.out.println("Dog barks");}}
Animal myAnimal = new Animal();Animal myCat = new Cat();Animal myDog = new Dog();
myAnimal.sound();// Output: "Animal makes a sound"myCat.sound();// Output: "Cat meows"myDog.sound();// Output: "Dog barks"```
In this example, even though the objects are declared as the superclass "Animal," the actual behavior executed is based on the specific subclass type.
Encapsulation
Encapsulation is the practice of hiding internal details and providing controlled access to an object's properties and methods. It helps maintain data integrity and protects the object from unwanted modifications. In Java, you can achieve encapsulation by defining private attributes and providing public methods (getters and setters) to access and modify those attributes.
```javaclass Person {private String name;public String getName() {return name;}public void setName(String newName) {name = newName;}}```
In this example, the "name" attribute is private, meaning it cannot be accessed directly from outside the class. Instead, the public getter and setter methods provide controlled access to the attribute.
Building User Interfaces with XML
Creating visually appealing and user-friendly interfaces is crucial for the success of any Android app. In this section, we will explore how XML (eXtensible Markup Language) can be used to design Android app interfaces.
Understanding XML Layout Files
In Android, layout files are written in XML and define the structure and appearance of app screens. Each screen is associated with a layout file, which contains a hierarchy of view elements and their properties.
XML offers a structured and intuitive way to describe UI elements and their attributes. You can specify the position, size, text, color, and other visual aspects of views using XML tags and attributes. Understanding the basic syntax and available tags is essential for designing effective app interfaces.
Creating Views and View Groups
Views are the fundamental building blocks of an Android app's UI. They represent interactive elements such as buttons, text fields, images, and more. You can create views in XML by using specific tags and attributes.
For example, to create a button, you can use the <Button>
tag:
```xml
In this example, the android:id
attribute assigns a unique identifier to the button, allowing you to reference it in your Java code. The android:layout_width
and android:layout_height
attributes determine the button's size, and the android:text
attribute sets the button's text.
View groups, such as <LinearLayout>
and <RelativeLayout>
, are used to organize and arrange multiple views within a layout. They provide different layout options, such as horizontal or vertical orientation, enabling you to create visually appealing and well-structured app interfaces.
Handling User Input
Android apps often require user input for various purposes, such as form submission or interaction with app features. XML allows you to define UI elements that capture user input and handle it appropriately.
For example, to create a text input field, you can use the <EditText>
tag:
```xml
In this example, the android:id
attribute assigns an identifier to the text input field. The android:hint
attribute displays a hint message inside the field, providing guidance to the user.
To handle user input in your Java code, you can access the UI elements by their assigned IDs and attach appropriate listeners to capture user actions, such as button clicks or text input changes.
Enhancing UI with Styles and Themes
Android provides styles and themes to define the visual appearance of UI elements consistently across your app. Styles specify the visual properties of a particular view or view group, while themes define the overall look and feel of the app.
You can define styles and themes in XML and apply them to the desired UI elements. This allows you to customize the colors, fonts, shapes, and other visual aspects of your app's UI, giving it a unique and polished look.
App Navigation and Activity Lifecycle
Effective app navigation and understanding the activity lifecycle are crucial for providing a seamless user experience in your Android apps. In this section, we will explore app navigation principles and explain how activities interact with users during their lifecycle.
Activity Navigation: Intents and Activities
Android apps often consist of multiple screens (activities) that users can navigate through. App navigation is typically achieved using intents, which are objects that facilitate communication between different components of an app.
To navigate from one activity to another, you create an intent and specify the target activity:
```javaIntent intent = new Intent(MainActivity.this, SecondActivity.class);startActivity(intent);```
In this example, the intent starts the SecondActivity
from the MainActivity
. You can pass data between activities using intent extras, allowing you to transfer information and maintain context as users navigate through your app.
Activity Lifecycle: Managing State and User Interactions
Activities in Android have a lifecycle consisting of different stages, such as creation, starting, pausing, resuming, and destroying. Understanding the activity lifecycle is crucial for managing state changes and handling user interactions appropriately.
For example, you can override lifecycle callback methods, such as onCreate
and onResume
, to perform initialization tasks or update UI elements when an activity is created or resumed.
By leveraging the activity lifecycle, you can save and restore the state of your app, handle system events, and provide a seamless user experience even when users navigate away from your app and return later.
Fragments: Multi-Screen UI and Reusability
Fragments, as mentioned earlier, are reusable UI components that can be combined to create multi-screen UIs. By using fragments, you can create dynamic and flexible app interfaces that adapt to different screen sizes and orientations.
Furthermore, fragments provide reusability, allowing you to share UI components across multiple activities or even within the same activity. This promotes modular code and reduces duplication, making your app more maintainable and scalable.
Working with Data: Storing and Retrieving Information
Most Android apps require the ability to store and retrieve data. In this section, we will explore various techniques for data management in Android apps, such as using SQLite databases, SharedPreferences, and content providers.
SQLite Databases: Structured Data Storage
SQLite is a lightweight and database management system built into Android. It provides a structured and efficient way to store and retrieve data in your app. By usingSQLite databases, you can create tables with columns and rows to organize your data effectively.
To work with SQLite databases in Android, you can utilize the SQLiteOpenHelper class. This class helps you create, upgrade, and manage your database. You can define the table structure using SQL statements and perform CRUD operations (Create, Read, Update, Delete) to manipulate the data stored in the database.
SharedPreferences: Simple Data Storage
If you need to store simple key-value pairs or small amounts of data, SharedPreferences provide a lightweight and convenient solution. SharedPreferences allow you to store primitive data types, such as integers, booleans, and strings, persistently.
To work with SharedPreferences, you can use the SharedPreferences class and its various methods, such as putInt(), getBoolean(), and getString(). You can create separate SharedPreferences files for different data sets and access them throughout your app.
Content Providers: Data Sharing and Security
Content providers facilitate data sharing between different apps and enforce security measures. Content providers act as a bridge between your app's data and other apps, allowing them to access and modify the data securely.
To create a content provider, you need to define a contract that specifies data types, URIs (Uniform Resource Identifiers), and other necessary information. You can then implement the content provider and define CRUD operations to interact with the data.
By using content providers, you can securely share data with other apps, enable data synchronization, and ensure data integrity by enforcing proper access controls.
Implementing Functionality with Java
Now that you have a solid understanding of Java programming and the basics of Android app development, it's time to implement functionality in your apps. This section will cover various aspects of implementing functionality using Java.
Handling User Input
One of the essential aspects of app functionality is handling user input effectively. Whether it's capturing text input, responding to button clicks, or processing gestures, understanding how to handle user input is crucial for creating interactive and engaging apps.
In Android, you can attach event listeners to UI elements to capture user actions. For example, you can set an OnClickListener on a button to perform a specific action when the button is clicked:
```javaButton myButton = findViewById(R.id.myButton);myButton.setOnClickListener(new View.OnClickListener() {@Overridepublic void onClick(View v) {// Perform action on button click}});```
By implementing appropriate event listeners and responding to user input, you can create apps that are intuitive and responsive to user actions.
Making API Calls
Many Android apps rely on data from web services and APIs (Application Programming Interfaces). Making API calls allows your app to fetch data, interact with external services, and provide dynamic content to users.
In Java, you can use various libraries and frameworks, such as Retrofit or Volley, to make API calls. These libraries offer convenient methods for sending requests, handling responses, and parsing data in different formats, such as JSON or XML.
By integrating API calls into your app, you can provide real-time information, enable user authentication, and offer features that depend on external data sources.
Integrating Third-Party Libraries
Android provides a rich ecosystem of third-party libraries and frameworks that can enhance your app's functionality and save development time. These libraries offer pre-built components, utilities, and tools that you can integrate into your app.
Before integrating a third-party library, ensure that it aligns with your app's requirements and has good community support. You can usually integrate libraries by adding their dependencies to your app's build.gradle file.
For example, if you want to add the popular Picasso library for image loading and caching:
```gradledependencies {implementation 'com.squareup.picasso:picasso:2.71828'}```
By leveraging third-party libraries, you can add advanced features, improve performance, and focus more on the unique aspects of your app's functionality.
Implementing Essential App Features
Every app has specific features and functionalities that make it unique. This section will explore some essential app features that you can implement in your Android apps, depending on your app's purpose and target audience.
Data Persistence and Offline Functionality
Implementing data persistence allows your app to save data locally, enabling users to access it even when they are offline. By storing data locally, you can enhance app performance, reduce network usage, and provide a seamless experience for users.
You can use the techniques mentioned earlier, such as SQLite databases or SharedPreferences, to persist data. Additionally, you can implement caching mechanisms to store frequently accessed data, reducing the need for repeated network requests.
User Authentication and Account Management
If your app requires user accounts or personalized experiences, implementing user authentication and account management features is essential. This allows users to create accounts, log in securely, and access personalized content or data.
Android provides various authentication mechanisms, such as Firebase Authentication, which offers ready-to-use authentication services. By integrating authentication into your app, you can secure user data, personalize the user experience, and enable data synchronization across devices.
Push Notifications and In-App Messaging
Push notifications and in-app messaging are powerful tools for engaging with users and providing timely updates or information. Implementing these features allows you to send notifications directly to users' devices, even when your app is not actively running.
Android provides Firebase Cloud Messaging (FCM) for implementing push notifications. By integrating FCM into your app, you can send targeted notifications, display custom messages, and drive user engagement.
Location-Based Services
Location-based services enable your app to leverage the device's GPS or network connectivity to provide location-specific information or functionality. This can include features such as mapping, geolocation, or location-aware recommendations.
Android offers the Google Maps API and other location-related APIs to implement location-based services. By integrating these APIs, you can access location data, display maps, and create location-aware experiences in your app.
Testing and Debugging Your Apps
Testing and debugging are crucial steps in the app development process to ensure your app functions smoothly and efficiently. This section will cover various testing techniques and debugging tools available for Android app development.
Unit Testing
Unit testing involves testing individual units or components of your app in isolation to ensure they function correctly. By writing unit tests, you can verify that specific methods or classes behave as expected and catch any potential bugs or issues.
Android provides frameworks, such as JUnit and Espresso, for writing and running unit tests. You can create test cases that simulate different scenarios and expected outcomes, allowing you to validate the behavior of your app's code.
Integration Testing
Integration testing focuses on testing how different components of your app work together and interact with each other. This type of testing ensures that the integration between various modules, APIs, or services is seamless and functioning as intended.
Android provides frameworks like Espresso and UI Automator for writing integration tests. These frameworks allow you to simulate user interactions, test UI elements, and verify the overall flow and functionality of your app.
Debugging Tools
Android Studio offers a variety of debugging tools to help you identify and fix issues in your app. The built-in debugger allows you to pause the app's execution at specific breakpoints, inspect variables, and step through the code line by line to understand its behavior.
The logcat window in Android Studio displays system logs, error messages, and debugging information, providing valuable insights into your app's behavior and potential issues. By analyzing logcat output, you can identify exceptions, performance bottlenecks, or unexpected behavior in your app.
Emulator and Device Testing
Testing your app on different devices and Android versions is crucial to ensure compatibility and optimal performance. Android Studio provides an emulator that allows you to simulate various device configurations and test your app's behavior on different screen sizes, resolutions, and hardware specifications.
In addition to emulator testing, it's essential to test your app on real devices to ensure accurate performance and user experience. You can connect physical devices to your development environment and run your app directly on them for thorough testing.
Publishing Your App to the Google Play Store
Congratulations! Your app is ready to be shared with the world. This section will guide you through the process of publishing your Android app on the Google Play Store, making it available to millions of users.
Preparing Your App for Release
Before publishing your app, you need to ensure that it meets certain criteria and follows the guidelines set by the Google Play Store. This includes optimizing your app's performance, ensuring it adheres to design principles, and addressing any potential security vulnerabilities.
Make sure to thoroughly test your app, fix any bugs or crashes, and optimize its performance. Pay attention to app compatibility across different devices and screen sizes, ensuring your app provides a consistent experience to all users.
Creating a Developer Account
To publish your app on the Google Play Store, you need to create a developer account. This involves providing necessary information, agreeing to the terms and conditions, and paying a one-time registration fee.
Visitthe Google Play Console website and follow the instructions to create your developer account. Once your account is set up, you will have access to the Play Console dashboard, where you can manage your app listings, track analytics, and release updates.
Preparing App Assets and Metadata
Before submitting your app to the Google Play Store, you need to prepare the necessary assets and metadata that will be displayed to users. This includes creating an app icon, writing a compelling app description, providing screenshots or promotional images, and selecting appropriate categories and tags.
Ensure that your app icon is visually appealing and represents your app's branding effectively. Write a concise and engaging app description that highlights the key features and benefits of your app. Capture screenshots or promotional images that showcase your app's UI and functionality, helping users understand what they can expect from your app.
Building a Release Version
To publish your app, you need to build a release version of your app package (APK file). A release version is optimized for distribution, has fewer debugging features, and is signed with a release key to ensure its authenticity.
In Android Studio, you can create a release version by selecting "Build" from the menu, then choosing "Generate Signed Bundle/APK." Follow the prompts to create a keystore file and sign your app with a release key. The generated APK file can then be uploaded to the Google Play Console.
Uploading and Publishing Your App
Once you have prepared your app assets, metadata, and the release version of your app, you can upload it to the Google Play Console. Sign in to your developer account and navigate to the "App releases" section.
Click on "Create Release" and follow the steps to upload your APK file, fill in the required details, and submit your app for review. The review process ensures that your app meets the Play Store's policies and guidelines. Once your app is approved, it will be published on the Google Play Store and available for users to download and install.
Remember to regularly update your app, address user feedback, and stay updated with the latest Android versions and features to provide the best possible experience to your users.
By following this comprehensive guide, you have gained the knowledge and skills necessary to embark on your Android app development journey. Remember, practice and continuous learning are key to mastering the art of Android app development. So, start implementing your ideas, explore new possibilities, and bring your unique app creations to life!
Happy coding!