How to Start Developing an Android App: A Comprehensive Guide
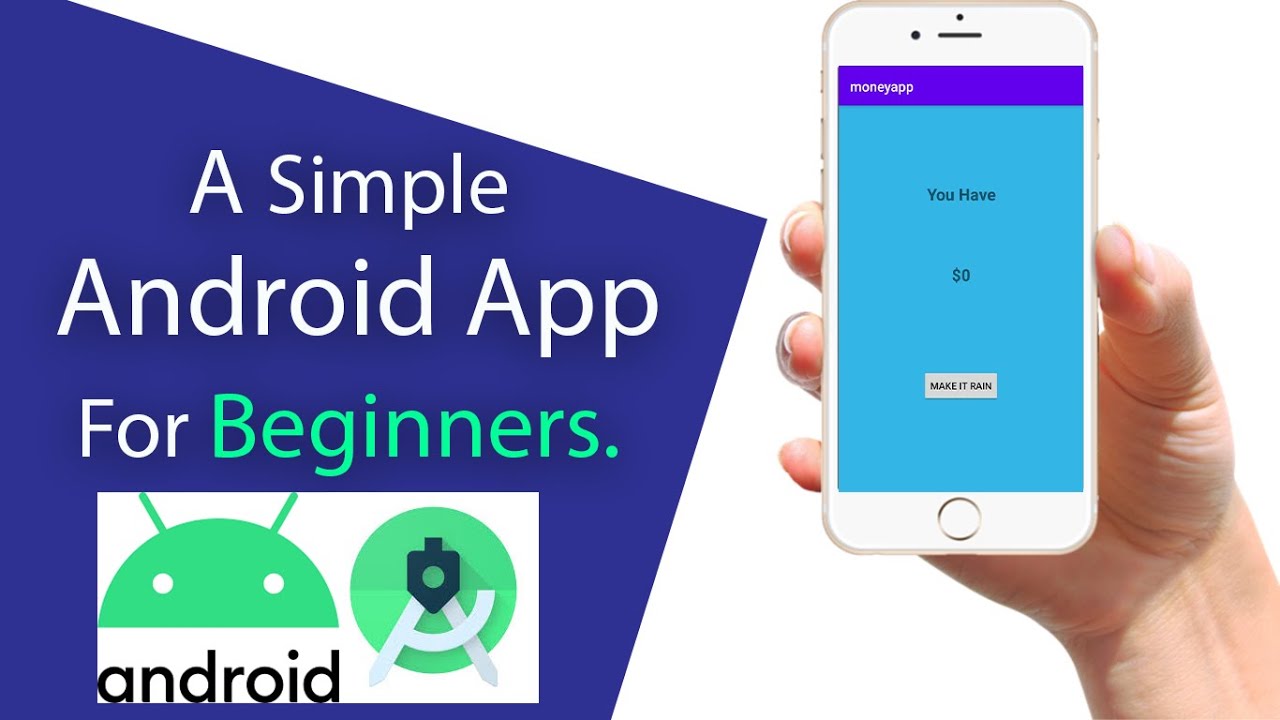
Are you eager to dive into the world of Android app development? Whether you're a beginner or an experienced programmer looking to expand your skillset, this comprehensive guide will help you get started on your journey. In this article, we will walk you through the essential steps and provide you with valuable insights to develop your own Android app from scratch.
Before we begin, it's important to note that developing an Android app requires some understanding of programming concepts and familiarity with Java or Kotlin. However, don't let that discourage you – with determination and the right guidance, you can turn your app development dreams into reality.
Understanding the Basics of Android App Development
In this section, we will cover the fundamental concepts of Android app development, including the Android operating system, the development environment, and the necessary tools you'll need to get started.
Android Operating System:
The Android operating system is an open-source platform developed by Google. It powers millions of devices worldwide and provides a robust framework for building mobile applications. Understanding the basics of the Android operating system will give you a solid foundation for app development.
Development Environment:
Android app development primarily takes place in an integrated development environment (IDE) called Android Studio. Android Studio provides a comprehensive set of tools and features specifically designed for developing Android apps. It streamlines the development process and offers powerful debugging capabilities.
Necessary Tools:
Before you start developing Android apps, you'll need to set up your development environment. This includes installing Android Studio, which comes bundled with the Android SDK (Software Development Kit). The SDK contains essential libraries, tools, and APIs necessary for building Android apps. Additionally, you'll need to install the Java Development Kit (JDK) to write code in Java or Kotlin.
Setting Up Your Development Environment
Before you can start building your Android app, you need to set up your development environment. This section will guide you through installing Android Studio, configuring the necessary SDKs, and setting up virtual devices for testing your app.
Installing Android Studio
The first step in setting up your development environment is to download and install Android Studio. Visit the official Android Studio website and download the latest stable version for your operating system. Once the download is complete, run the installer and follow the on-screen instructions to install Android Studio.
Configuring the SDKs
After installing Android Studio, you'll need to configure the necessary SDKs. The SDK Manager in Android Studio allows you to download different versions of the Android SDK, including the latest platform tools and system images for testing on different device configurations. Open the SDK Manager and select the desired SDK components to download and install.
Setting Up Virtual Devices
To test your app on different devices without physically owning them, you can use virtual devices provided by the Android Emulator. The Android Emulator allows you to simulate various device configurations and screen sizes. In Android Studio, you can create and manage virtual devices through the AVD Manager. Create a new virtual device and select the desired device configuration, such as screen size, resolution, and Android version.
Getting to Know Android Studio
Now that your development environment is ready, it's time to familiarize yourself with Android Studio. This section will introduce you to the various components of Android Studio, such as the project structure, the layout editor, and the code editor.
Project Structure
When you open Android Studio, you'll be greeted with the project structure. The project structure consists of different directories and files that organize your app's code and resources. Understanding the project structure is essential for efficient navigation and management of your app's files.
Layout Editor
The layout editor in Android Studio allows you to visually design your app's user interface. It provides a drag-and-drop interface where you can add UI components, arrange them on the screen, and customize their properties. The layout editor generates XML code behind the scenes, which defines the structure and appearance of your app's UI.
Code Editor
The code editor in Android Studio is where you write the actual code for your app. It supports multiple programming languages, including Java and Kotlin. The code editor provides features like syntax highlighting, code completion, and code analysis, making it easier to write clean and efficient code.
Understanding the Android Application Architecture
Before writing code, it's crucial to understand the architecture of an Android application. This section will explain the key components of an Android app, such as activities, layouts, and resources, and how they work together to create a functional app.
Activities
An activity is a single screen in an Android app. It represents a user interface with which the user can interact. Understanding the lifecycle of an activity is crucial for managing the state of your app and handling user interactions effectively.
Layouts
A layout defines the structure and appearance of an activity's user interface. It acts as a container for UI components, such as buttons, text views, and images. Android provides different types of layouts, such as LinearLayout, RelativeLayout, and ConstraintLayout, each offering different ways to organize and align UI components.
Resources
Resources in Android refer to non-code assets used by your app, such as images, strings, and dimensions. By separating resources from code, you can easily customize your app's appearance and support multiple languages and device configurations. Understanding how to access and utilize resources is essential for creating a dynamic and adaptable app.
Designing Your App's User Interface
The user interface (UI) plays a vital role in the success of your app. In this section, we will discuss the principles of UI design and guide you through creating visually appealing and user-friendly interfaces using XML and the layout editor in Android Studio.
Principles of UI Design
Effective UI design is crucial for ensuring a positive user experience. This subheading will cover key principles of UI design, such as simplicity, consistency, and responsiveness. By understanding these principles, you'll be able to create intuitive and visually appealing interfaces for your app.
Creating UI Components with XML
In Android, UI components are defined using XML (eXtensible Markup Language). XML allows you to describe the structure and properties of UI elements in a hierarchical manner. This subheading will guide you through writing XML code to create UI components, setting attributes, and handling events.
Using the Layout Editor
The layout editor in Android Studio provides a visual interface for creating UI components. This subheading will show you how to use the layout editor to drag and drop UI elements onto the screen, arrange them, and customize their properties. The layout editor generates XML code in the background, allowing you to switch between visual and code editing seamlessly.
Implementing Functionality with Java/Kotlin
Now it's time to add functionality to your app! This section will cover the basics of programming in Java or Kotlin, including variables, control flow, and object-oriented programming. You'll learn how to write code to handle user interactions, perform calculations, and more.
Introduction to Java/Kotlin
If you're new to Java or Kotlin, this subheading will provide a brief introduction to the programming language you'll be using for Android app development. It will cover basic syntax, data types, and control structures, giving you a solid foundation to start writing code.
Handling User Interactions
Most apps require user interactions, such as button clicks or text input. This subheading will show you how to handle user interactions in your app by setting up event listeners and responding to user actions. You'll learn how to write code that executes when a button is clicked or when the user enters text in an input field.
Performing Calculations and Data Manipulation
Many apps involve calculations or data manipulation. This subheading will teach you how to write code to perform calculations, manipulate data, and store information in variables. You'll learn about arithmetic operations, string manipulation, and data structures like arrays and lists.
Working with Data: Storing and Retrieving Information
Many apps require data storage capabilities. In this section, we will explore different options for storing and retrieving data in your Android app, including shared preferences, SQLite databases, and content providers.
Shared Preferences
Shared preferences allow you to store small amounts of data as key-value pairs. This subheading will show you how to use shared preferences to store user preferences, settings, or other simple data that needs to persist across app sessions.
SQLite Databases
If your app needs to store larger amounts of structured data, SQLite databases provide a powerful solution. This subheading will guide you through creating a SQLite database, defining tables and columns, and performing CRUD (Create, Read, Update, Delete) operations on the data.
Content Providers
Content providers allow you to securely share data between different apps or access system data. This subheading will explain how to create a content provider, define data URIs, and implement query, insert, update, and delete operations.
Testing and Debugging Your App
Testing and debugging are critical steps in the app development process. This section will guide you through the different testing methodologies, including unit testing and UI testing, and show you how to use Android Studio's debugging tools to identify and fix issues.
Unit Testing
Unit testing involves testing individual units or components of your app in isolation. This subheading will introduce you to the concept of unit testing and show you how to write test cases using frameworks like JUnit. You'll learn how to verify the correctness of your code and ensure that individual units function as expected.
UI Testing
UI testing focuses on testing the user interface of your app. This subheading will cover UI testing frameworks like Espresso, which allows you to write automated tests to simulate user interactions and verify the behavior of your app's UI components. You'll learn how to write UI test cases and run them using Android Studio's testing tools.
Debugging Your App
Debugging is the process of identifying and resolving issues in your app's code. This subheading will show you how to use Android Studio's debugging tools, such as breakpoints, watches, and logcat, to diagnose and fix bugs in your app. You'll learn how to step through code, inspect variables, and analyze runtime errors to improve the quality of your app.
Publishing Your App to the Google Play Store
Once your app is ready, it's time to share it with the world! In this section, we will walk you through the process of preparing your app for release, generating a signed APK, and submitting it to the Google Play Store.
Preparing Your App for Release
Before you can publish your app, you need to ensure that it meets certain requirements and guidelines set by the Google Play Store. This subheading will cover steps like optimizing your app's performance, testing on different devices, and addressing any security vulnerabilities. You'll learn how to make your app ready for a smooth release.
Generating a Signed APK
To publish your app on the Google Play Store, you need to generate a signed APK (Android Package Kit). This subheading will guide you through the process of signing your app, which involves creating a keystore, configuring build settings, and generating a signed APK file that can be uploaded to the Google Play Console.
Submitting to the Google Play Store
Once you have a signed APK, you're ready to submit your app to the Google Play Store. This subheading will walk you through the steps to create a developer account, create a store listing for your app, upload the APK, and set pricing and distribution options. You'll learn how to provide app screenshots, descriptions, and other assets to make your app appealing to potential users.
Continuing Your Learning Journey
App development is an ever-evolving field, and there's always more to learn. In this final section, we will provide you with resources and recommendations to further enhance your Android app development skills and stay up-to-date with the latest trends and technologies.
Online Tutorials and Documentation
One of the best ways to continue learning is by exploring online tutorials and documentation. This subheading will provide you with recommendations for websites, blogs, and online courses where you can find in-depth tutorials, code samples, and documentation on various aspects of Android app development.
Communities and Forums
Engaging with the developer community can greatly enrich your learning experience. This subheading will introduce you to popular online communities and forums where you can connect with fellow developers, ask questions, share your knowledge, and stay updated with the latest news and discussions in the Android development community.
Attend Conferences and Workshops
Attending conferences and workshops related to Android app development can provide valuable networking opportunities and insights into the latest industry trends. This subheading will highlight some well-known conferences and workshops where you can learn from experts, participate in hands-on sessions, and gain inspiration for your future app projects.
Contributing to Open-Source Projects
Contributing to open-source projects allows you to collaborate with other developers and contribute to the Android community. This subheading will encourage you to explore open-source projects on platforms like GitHub and provide guidance on how to get started with contributing, whether it's through code contributions, documentation, or bug fixes.
In conclusion, developing an Android app may seem daunting at first, but with the right guidance and a passion for learning, you can embark on this exciting journey. By following this comprehensive guide, you'll gain a solid foundation in Android app development and be well-equipped to bring your app ideas to life. So, roll up your sleeves, fire up Android Studio, and let's get started!