Create an Android App from Scratch: A Comprehensive Guide
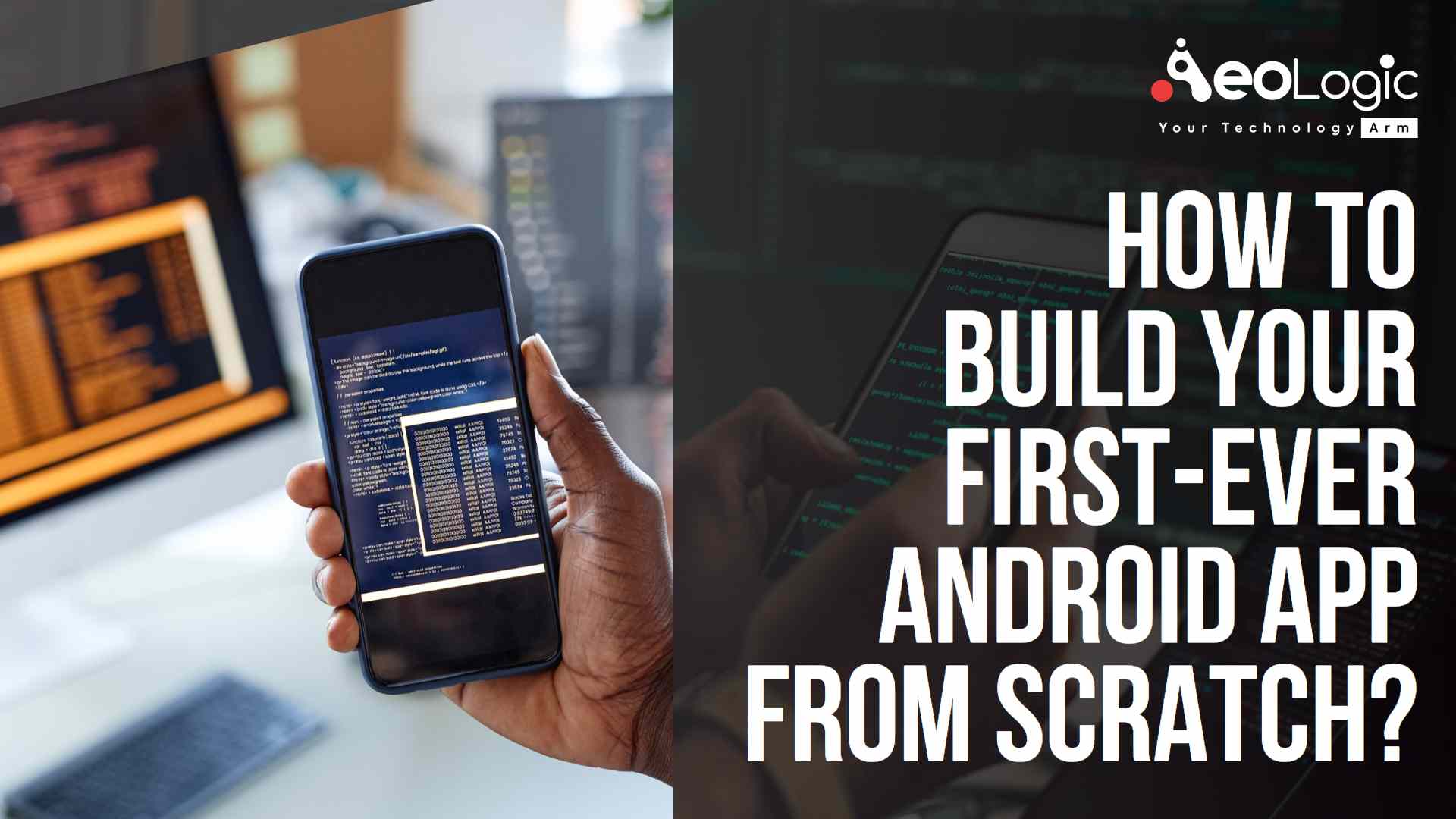
Are you interested in creating your own Android app from scratch but don't know where to start? Look no further! In this comprehensive guide, we will walk you through the step-by-step process of building an Android app from the ground up. Whether you're a beginner or have some coding experience, this article will provide you with the knowledge and resources you need to develop a fully functional app.
Before we dive into the nitty-gritty details, let's first understand what it means to create an Android app from scratch. Building an app from scratch refers to starting with a blank canvas and writing every line of code and designing every element of the app yourself. This gives you full control over the app's functionalities, appearance, and overall user experience.
Planning Your App
In this section, we will discuss the importance of planning your app before diving into development. Effective planning is crucial as it sets the foundation for a successful app. By defining the purpose of your app, you can ensure that it solves a problem or meets a need in the target market. Identify your target audience and conduct thorough market research to understand their preferences, pain points, and expectations. This will help you tailor your app to their specific needs.
Defining the Purpose of Your App
Before you start designing and coding your app, it's essential to have a clear understanding of its purpose. Ask yourself, "What problem does my app solve?" or "What need does it fulfill?" This will guide you in creating an app that provides value to its users. Whether it's a productivity tool, a social networking platform, or a gaming app, defining the purpose will help you stay focused and deliver a cohesive user experience.
Identifying Your Target Audience
Understanding your target audience is key to building a successful app. Conduct market research to identify the demographics, preferences, and behaviors of your potential users. This information will guide your design and development decisions, ensuring that your app resonates with its intended audience. Consider factors such as age, gender, location, and interests to create an app that meets their specific needs.
Creating a Wireframe
A wireframe is a visual representation of your app's structure and layout. It acts as a blueprint for your app, allowing you to visualize its flow and organization. Use wireframing tools or sketch on paper to create a rough outline of your app's screens, navigation, and interactions. This will help you identify any usability issues or gaps in functionality early on, saving time and effort during development.
Setting Up Your Development Environment
Before you can start coding, you need to set up your development environment. This section will guide you through the installation and configuration of necessary tools such as Android Studio, the official Integrated Development Environment (IDE) for Android app development. We will also cover setting up an emulator or connecting a physical device for testing your app.
Installing Android Studio
Android Studio is the go-to IDE for Android app development. It provides a comprehensive set of tools and features to streamline the development process. To install Android Studio, visit the official Android Developer website and download the latest version for your operating system. Follow the installation instructions provided by the Android Studio installer. Once installed, open Android Studio and configure the necessary settings to customize your development environment.
Setting Up an Emulator
An emulator allows you to test your app on virtual Android devices without the need for a physical device. Android Studio provides an emulator that simulates various device configurations and screen sizes. To set up an emulator, open Android Studio and navigate to the AVD (Android Virtual Device) Manager. Create a new virtual device by selecting the device type, screen size, and other specifications. Once created, you can launch the emulator and test your app on different virtual devices.
Connecting a Physical Device
If you prefer testing your app on a physical device, you can connect it to your development computer via USB. Ensure that USB debugging is enabled on your device by going to the Developer Options in the device settings. Once enabled, connect your device to your computer using a USB cable. Android Studio will recognize the device, and you can select it as the target device for running and testing your app.
Understanding Java Basics for Android Development
Java is the primary programming language used for Android app development. In this section, we will provide an in-depth overview of Java basics, including object-oriented programming concepts, variables, data types, and control structures. This knowledge will serve as the foundation for writing code in Android Studio.
Introduction to Java
If you're new to Java, it's essential to understand its key concepts and syntax. Java is an object-oriented programming language that allows you to organize your code into reusable objects. Objects have attributes (variables) and behaviors (methods) that define their characteristics and actions. Familiarize yourself with Java's syntax, including how to declare variables, define classes, and write methods.
Object-Oriented Programming in Java
Object-oriented programming (OOP) is a programming paradigm that focuses on organizing code into objects that interact with each other. In Java, OOP principles such as encapsulation, inheritance, and polymorphism are essential for building robust and maintainable apps. Learn how to create classes, define relationships between classes through inheritance, and utilize interfaces to implement polymorphism.
Variables and Data Types
In Java, variables are used to store data that can be manipulated and accessed throughout your app. Understand the different data types in Java, such as integers, floating-point numbers, booleans, and strings. Learn how to declare variables, assign values, and perform operations on them. Additionally, explore more complex data structures like arrays and collections for storing and manipulating larger sets of data.
Control Structures and Flow Control
Control structures allow you to control the flow of execution in your app. Learn about conditional statements like if-else and switch-case to make decisions based on certain conditions. Dive into loop structures like for, while, and do-while to repeat certain actions multiple times. Understanding control structures is crucial for implementing logic and making your app respond dynamically to user interactions or data changes.
Designing the User Interface (UI)
The user interface is a crucial aspect of any app. In this section, we will explore the tools and techniques available in Android Studio to design an intuitive and visually appealing UI. We will cover XML layout files, UI components such as buttons and text fields, and how to handle user interactions. By the end of this section, you will have a well-designed UI for your app.
Understanding Layouts and Views
In Android, layouts are used to define the structure and arrangement of UI elements. Learn about different types of layouts, such as LinearLayout, RelativeLayout, and ConstraintLayout, and when to use each one. Familiarize yourself with various UI views like TextView, Button, ImageView, and EditText, and how to customize their appearance and behavior.
Creating XML Layout Files
XML layout files are used to define the UI of your app. Learn how to create XML layout files in Android Studio and use the available layout and view attributes to customize the appearance and positioning of UI elements. Understand the concept of view hierarchy and how to nest layouts to achieve complex UI designs.
Handling User Input
User input is an essential aspect of many apps. Learn how to handle user interactions, such as button clicks, text input, and gestures. Implement event listeners in your code to capture user actions and respond accordingly. Validate user input and provide appropriate feedback to ensure a smooth and error-free user experience.
Working with Fragments
Fragments are modular components in Android that encapsulate UI and logic. They are used to create reusable UI elements and facilitate flexible screen layouts. Learn how to work with fragments to create dynamic and responsive UIs. Understand fragment lifecycle and how to communicate between fragments to exchange data or trigger actions.
Implementing App Functionality
Now that you have a solid foundation in Java and a well-designed UI, it's time to implement the core functionality of your app. This section will cover topics such as handling user input, making API requests, saving data locally, and integrating third-party libraries. By the end of this section, your app will have the necessary features to provide a valuable user experience.
Handling User Input and Events
User input is the primary method of interacting with your app. Learn how to handle user input events such as button clicks, touch gestures, and text input. Implement event listeners and callbacks to capture user actions and trigger appropriate actions or updates in your app. Validate and process user input to ensure data integrity and provide meaningful feedback.
Making API Requests
Many apps require data from external sources, such as web APIs. Learn how to make HTTP requests to fetch data from remote servers and integrate it into your app. Understand different request methods like GET, POST, PUT, and DELETE, and how to handle API responses. Implement libraries like Retrofit or Volley to simplify the process of making API requests and handling data parsing.
Saving and Retrieving Data Locally
Storing data locally can enhance your app's performance and allow offline functionality. Learn how to save and retrieve data usinglocal storage options in Android, such as SharedPreferences, SQLite databases, or file storage. Understand the pros and cons of each method and choose the most suitable one for your app's data requirements. Implement data persistence to store user preferences, app settings, or cached data for efficient and seamless user experience.
Integrating Third-Party Libraries
Third-party libraries can significantly speed up development and provide additional functionality to your app. Learn how to integrate popular libraries into your Android project. Research and choose libraries that fulfill specific needs, such as image loading, networking, UI animation, or database management. Understand the process of adding library dependencies to your project, configuring them, and utilizing their features in your code.
Testing and Debugging Your App
No app is complete without thorough testing and debugging. In this section, we will discuss various testing strategies and tools available in Android Studio to ensure your app works as intended. We will cover unit testing, UI testing, and debugging techniques to identify and fix any issues that may arise during development.
Unit Testing
Unit testing is a crucial aspect of app development to ensure that individual units of code work correctly. Learn how to write unit tests for your app's classes and methods using frameworks like JUnit or Mockito. Understand the importance of test-driven development (TDD) and how to create test cases that cover different scenarios and edge cases. Execute unit tests regularly to catch bugs early and maintain code quality.
UI Testing
UI testing is essential to verify that your app's user interface behaves as expected across different devices and screen sizes. Learn how to write UI tests using frameworks like Espresso or UI Automator. Create automated test scripts that simulate user interactions and verify the correctness of UI elements, navigation, and data display. Run UI tests on emulators or physical devices to ensure consistent user experiences.
Debugging Techniques
Debugging is a crucial skill for any developer. Learn how to effectively use the debugging tools provided by Android Studio to identify and fix issues in your app. Understand how to set breakpoints, inspect variables, step through code execution, and analyze stack traces. Utilize logging statements to track the flow of your app and identify potential bugs or performance bottlenecks. Debugging will help you isolate and resolve issues, ensuring a stable and reliable app.
Publishing Your App to the Google Play Store
Once your app is ready for the world to see, you'll need to publish it to the Google Play Store. This section will guide you through the process of creating a developer account, preparing your app for release, and submitting it to the Play Store. We will also cover tips for optimizing your app's visibility and increasing downloads.
Creating a Developer Account
To publish your app on the Google Play Store, you'll need to create a developer account. Follow the steps provided by Google to create an account and pay the one-time registration fee. Provide the necessary information, such as your app's name, description, screenshots, and promotional materials. Familiarize yourself with the Play Store's policies and guidelines to ensure your app meets the requirements for publication.
Preparing Your App for Release
Before submitting your app, ensure that it is thoroughly tested and free of any bugs or issues. Optimize your app's performance, responsiveness, and battery usage. Use the Play Store's App Pre-Launch Report to identify potential issues on different devices and configurations. Prepare the necessary assets, such as app icons, promotional images, and a compelling app description. Consider localizing your app to reach a broader audience.
Submitting Your App
When you're ready to publish, create a listing for your app on the Play Store's Developer Console. Provide all the required information, including app title, description, screenshots, and categorization. Set the appropriate pricing and distribution options for your app. Upload the app's APK file, ensuring it meets the size and compatibility requirements. Review and submit your app for review by the Play Store team.
Optimizing App Visibility and Downloads
After publishing your app, it's essential to optimize its visibility to increase its chances of being discovered and downloaded by users. Understand the principles of App Store Optimization (ASO) and implement strategies to improve your app's ranking in search results. Encourage user reviews and ratings to build social proof and credibility. Consider promoting your app through digital marketing channels, social media, or collaborations with influencers to reach a wider audience.
Maintaining and Updating Your App
Building an app is just the beginning. In this section, we will discuss the importance of maintaining and updating your app to ensure it stays relevant and bug-free. We will cover strategies for collecting user feedback, monitoring app performance, and releasing updates to address issues or introduce new features.
Collecting User Feedback
Feedback from users is invaluable for improving your app's user experience and addressing any issues or shortcomings. Implement mechanisms to collect feedback, such as in-app surveys, ratings prompts, or user support channels. Actively listen to user suggestions and bug reports, and consider incorporating their feedback into your app's roadmap. Engage with your users and foster a community around your app for continuous improvement.
Monitoring App Performance
Regularly monitoring your app's performance is essential to identify any anomalies, crashes, or performance bottlenecks. Utilize tools like Firebase Crashlytics or Google Analytics to track app crashes, user engagement, and performance metrics. Analyze the data to identify trends, prioritize bug fixes or optimizations, and make informed decisions for future updates. Monitoring app performance ensures a smooth and reliable experience for your users.
Releasing Updates
To keep your app relevant and competitive, it's crucial to release regular updates with new features, bug fixes, and improvements. Gather user feedback, prioritize feature requests, and plan your updates accordingly. Follow a release management process to ensure smooth updates, including alpha and beta testing phases. Communicate with your users about the updates through release notes and changelogs. Regularly analyze user feedback and app performance data to iterate and enhance your app over time.
Resources and Further Learning
To become a proficient Android app developer, continuous learning is essential. In this section, we will provide a curated list of resources, including books, online tutorials, and communities, to help you further enhance your skills and stay up-to-date with the latest trends in Android app development.
Books and Online Tutorials
Explore recommended books and online tutorials that cover various aspects of Android app development. These resources will provide you with in-depth knowledge and practical examples to deepen your understanding of the platform and its best practices. Choose resources based on your skill level and specific areas of interest, such as UI design, database management, or advanced topics like performance optimization or security.
Communities and Forums
Joining Android developer communities and forums can greatly enrich your learning experience. Engage with fellow developers, ask questions, and share your knowledge. Participate in online discussions or attend local meetups to network with professionals in the field. These communities often share valuable insights, code samples, and industry news that can keep you informed and inspired in your Android app development journey.
Online Courses and Certifications
Consider enrolling in online courses or pursuing certifications to gain formal recognition and structured learning in Android app development. Platforms like Udemy, Coursera, or Google's Android Training offer a wide range of courses tailored to different skill levels and topics. Completing these courses and earning certifications can enhance your resume and demonstrate your expertise to potential employers or clients.
Conclusion
Creating an Android app from scratch may seem like a daunting task, but with the right guidance and resources, it's an achievable goal. By following the steps outlined in this comprehensive guide, you can bring your app idea to life and join the ever-growing community of Android developers. Remember, persistence and a willingness to learn are key to success in the exciting world of app development. So, let's get started and turn your app dreams into reality!